Advanced C++ Programming
Course
Inhouse
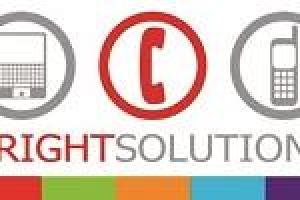
Description
-
Type
Course
-
Methodology
Inhouse
-
Start date
Different dates available
This course broadens the skills of a C++ language programmer by examining sophisticated C++ concepts such as templates, exceptions, memory management, advanced inheritance issues, disambiguation of overloaded functions, private and protected inheritance, binary i/o and class libraries.ObjectivesAt the end of this course, students will be able to:Decide between global functions, friend functions and member functionsCode their own memory management routines by overloading operators new and deleteWrite classes and functions with parameterized typesUnderstand and handle exceptions in C++ programsDisambiguate data and functions using multiple inheritanceUnderstand the difference between various kinds of inheritanceUse pointers to class member functionsUnderstand the C++ mechanism to resolve overloaded functions
Facilities
Location
Start date
Start date
About this course
TopicsA ReviewParameterized Types – TemplatesRelations of All KindsMultiple InheritanceData StructuresFunction PointersExceptionsThe C++ Standard Template LibraryDisambiguationFile I/OMiscellaneous Topics
Anybody who has been programming in C++ and wishes to enhance their knowledge of the language
Students should have completed the Introduction to C++ Programming course or have equivalent knowledge.
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 6 years
Subjects
- Inheritance
- C programming
- C language
- Composition
- Private
- Programming
Course programme
#text-block-10 { margin-bottom:0px; text-align:left; }
1. What You Should Already Know – A Review
Rationale for a new programming paradigm
The language of Object Orientation
A typical C++ class – a string class
Issues regarding member functions
member vs non member function
friend vs non friend
functions – returning references
Why does operator= return a reference?
Relationships
Simple C++ I/O
The uses of const
2. Parameterized Types – Templates
Templates
Overloading functions
Template functions
Specializing a template function
Disambiguation under specialization
Template classes
An array template class
Instantiating a template class object
Rules for templates
Non member function w/ a template argument
Friends of template classes
Templates with multiple type parameters
Comments regarding templates
3. Relations of All Kinds
Uses of Member Initialization Lists
Initialization lists under composition
Initialization lists under inheritance
Initialization lists w/ Multiple Inheritance (MI)
Initialization with MI and composition
Efficiency
operator= and composition
Constructors and composition
What is not inherited?
operator=, construction, and inheritance
Designing for inheritance
Public inheritance
Simple inheritance
Virtual functions
A shape class hierarchy
Polymorphism
Pure virtual functions
Virtual destructors
Abstract base classes
Private inheritance
Private inheritance vs composition
Using relationships
Associations
4. Multiple Inheritance
Multiple inheritance
Ambiguities
Removing ambiguities
virtual base classes
virtual base classes and the dominance rule
Member initialization lists with MI
operator= and MI
Designing for inheritance
5. Data Structures
Introduction
A simple List
Implementation of the list functions
Layering type safe classes upon List
A template List class
Iterators
A template iterator
Stack and Queue classes
A derived template array class
6. Function Pointers
Why have function pointers?
Passing functions as arguments
Registering functions
Callback functions
A class with a callback object
Registration of exceptions handlers
#text-block-11 { margin-bottom:0px; text-align:left; }
7. Exceptions
What are exceptions?
Traditional approaches to error handling
try, catch, and throw
A simple exception handler
Multiple catch blocks
The exception specification list
Rethrowing an exception
Cleanup
Exception matching
Inheritance and exceptions
Resource allocation
Constructors and exceptions
Destructors and exceptions
Catch by reference
Standard exceptions
8. The C++ Standard Template Library
History and evolution
New features
The Standard Template Library
STL Components
Iterators
Example: vector
Example: list
Example: set
Example: map
Example: find
Example: merge
Example: accumulate
Function objects
Adaptors
9. Disambiguation
Conversion
int Conversions
float
Arithmetic and pointer conversions
Inheritance based conversion
Overloaded functions
Exact match
Match with promotion
Match with standard conversion
User defined conversion
Constructors as conversion operators
Ambiguities
10. File I/O
Introduction
Error checking
Overloading << and >>
Formatted I/O
Disk files
Examples of seekg, tellg, and close
Reading and writing objects to the disk
Internal transmission of data
A larger I/O example: Spell checker
Treating a file as an array
11. Miscellaneous Topics
New features in the standard C++ language
Namespaces
Use counts
Run Time Type Identification
New casts
Overloading operator new and delete
How virtual functions are implemented
Having a limited number of objects
Smart pointers
Advanced C++ Programming