C++ Programming for C Programmers
Course
Inhouse
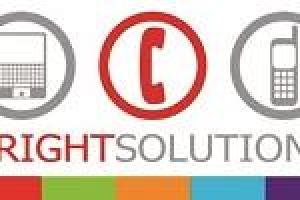
Description
-
Type
Course
-
Methodology
Inhouse
-
Start date
Different dates available
This course is a comprehensive hands-on introduction to object oriented programming in C++ for C programmers. Emphasis is placed on the features of C++ that support effective modeling of the problem domain and reuse of code. The course consists of two modules. In the first module object oriented concepts are introduced. The C++ class construct is introduced and its key features elaborated step-bystep, providing full implementation of abstract data types. C++ memory management is discussed. Function and operator overloading and the use of references are covered. The scope and access control mechanisms of C++ are described. Inheritance is introduced. The use of virtual functions to implement polymorphism is discussed. The second module presents intermediate topics. The ANSI C++ Standard Library is covered, including namespaces, the new header files and basic string class. Templates are covered, including an introduction to the Standard Template Library (STL).
Facilities
Location
Start date
Start date
About this course
C programmers who are moving to object oriented programming in C++.
A good working knowledge of C programming.
There is a chapter on I/O streams, including formatting and file I/O. Practical issues of C++ programming, such as reliability, testing, efficiency and interfacing to C, are discussed. The course introduces newer features of C++ such as exceptions, runtime type information (RTTI), and the new C++ cast syntax. Extensive programming examples and exercises are provided. The course is designed so that it can be taught in any environment with an ANSI C++ compiler.
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 6 years
Subjects
- Inheritance
- Access Control
- Access
- Object oriented training
- C programming
- Object-oriented training
- Programming
- Object oriented Programming
- Oriented Programming
Course programme
#text-block-10 { margin-bottom:0px; text-align:left; }
1. Concepts of Object-Oriented Programming
Object
State and Behavior
Abstraction
Encapsulation
Class and Instantiation
Abstract Data Types
Methods
Invoking Methods
Messages
Class Inheritance
Polymorphism
2. Classes in C++
Data Encapsulation in C
The C++ Class
Structures and Class in C++
Implementation of a C++ Class this Pointer
Code Organization
Scope Resolution Operator
Abstract Data Types
Test Programs for C++ Classes
3. Functions in C++
Function Prototypes in C++
Strong Type Checking
Conversion of Parameters
Inline Functions
Inline Functions in Header Files
Default Arguments
Function Overloading
Argument Matching
Argument Matching through Promotion
Match through Type Conversion
Call by Value
4. Constructors and Destructors
The Problem of Initialization
Constructors and Initialization
Constructor in Stack Class
Object Creation and Destruction
Destructors
Multiple Constructors
String Class Implementation
Hidden Constructors
Using a Default Argument
5. Memory Management in C++
Why is Memory Management Important in C++?
Choices for an Object’s Memory
Typical Memory Layout
Free Store Allocation
new Operator
Memory Allocation Errors new vs. malloc
delete Operator
Destructor (Review)
Hiding Memory Management
String Class Specification (Version 2)
String Class Implementation
String Class Bug
6. References and Argument Passing in C++
Variables
Argument Passing
Call-by-Value
Reference Declarations
Call-by-Reference
Copy Constructor
Default Copy Constructor
Bug in String Class
Specification of String Class
Implementation of String Class
Test Program
Output of Test Program
Review of Constant Types
Constants and Arguments
Chains of Functions Calls
const Objects and Member Functions
7. Operator Overloading, Initialization, and Assignment
Operator Overloading
Operator Functions
Semantics of return
Returning a Temporary Object
Returning a Reference
Initialization vs. Assignment
Semantics of Assignment Assignment
Assignment Bug
Overloading =
Review of this Pointer
Type Conversions
Conversion by Construction
Overloading Cast Operator
Test Program
8. Scope and Access Control
Scoping in C++
Block and Function Scope
File and Global Scope
Class Scope
Constant Types and Scope
Enumeration Types
Enumeration Types and Class Scope :: for Global Data
Static Class Members
Initialization of Static Member
Static Function Class Members
Access Control
Friend Functions
Invoking Member and Friend Functions
Implementing a Friend Function
Efficiency and Friend Functions
#text-block-11 { margin-bottom:0px; text-align:left; }
9. Introduction to Inheritance
Inheritance Concept
Inheritance in C++
Employee Test Program
Protected Members
Best Class Initializer List
Composition Member
Initializer List
Order of Initialization
Inheritance vs. Composition
10. Polymorphism and Virtual Functions
A Case for Polymorphism
Dynamic Binding
Pointer Conversion in Inheritance
Polymorphism Using Dynamic Binding
Virtual Function Specification
Invoking Virtual Functions
Vtable Virtual Destructors
Abstract Class Using Pure Virtual Function
Employee as an Abstract Class
Heterogeneous Collections
11. ANSI C++ Library
ANSI C++ Library
Hello ANSI C++
Namespaces
ANSI C++ String Class
Templates
12. Templates
General Purpose Functions
Macros
Function Templates
Template Parameters
Template Parameter Conversion
Generic Programming
General Purpose Classes
Class Templates
Array Class Implementation (array.h)
Using the Array Template
Template Parameters
Class Template Instantiation
Non Type Parameter Conversion
Standard Template Library
STL Components
STL Elements of a Simple Program
Simple STL Program
Map Container
13. Input/Output in C++
Input/Output in C++
Built-in Stream Objects
Output Operator <<
Input Operator >>
Character Input
String Input
Formatted I/O
Streams Hierarchy (Simplified)
File I/O
File Opening
Integer File Copy
Character File Copy
Overloading Stream Operators
Implementing Overloaded Stream Operators
14. Practical Aspects of C++ Programming
Interfacing C++ to Other Languages
Calling C from C++
_cplusplus Macro
Calling C++ from C
Interface Module for Stack Class
Namespace Collisions
ANSI Namespace
Reliability Philosophies of Languages
Prototypes and Type Checking
Constant Types
Access Control in C++
Reviews and Inspections
Inspections and C++
Testing Strategies for C++
Performance Considerations
Class Libraries
15. Exception Handling
Exception Handling
try and catch
Exception Flow of Control
Context and Stack Unwinding
Handling Exception in Best Context
Benefits of Exception Handling
Unhandled Exceptions
Clean Up
Multiple Catch Handlers
16. Runtime Type Information
Runtime Type and Polymorphism
type_info Class
typeid Operator
Compiler Options
Safe Pointer Conversions
Dynamic Cast
New C++ Style Casts
Static Cast
Reinterpret Cast
Const Cast
C++ Programming for C Programmers