Learning Path: Spring and Spring Boot Projects
Course
Online
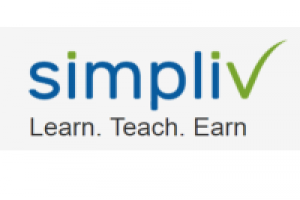
Description
-
Type
Course
-
Methodology
Online
-
Start date
Different dates available
Trying to build a highly robust application usually drifts the focus on infrastructure than functionality. Spring MVC and Spring Boot are the light-weight framework that comes to the rescue. This course will get you started with creating, deploying, and running a Spring MVC project using the Spring Tool Suite and will move on to building a Spring Boot application. At the end of the course, you will even get a glimpse of Spring security.About the AuthorKoushik KothagalKoushik Kothagal is the founder of Java Brains, an online training website that offers courses on various enterprise Java and JavaScript technologies entirely for free. He works as a Senior Staff Engineer at Financial Engines. He has over 14 years of professional experience working on full-stack web applications and has worked extensively with technologies such as Java, Spring, Java EE, JavaScript, and Angular. He loves teaching, and when he's not coding Java and JavaScript, he's probably teaching it! He currently lives in the Bay Area.Greg L. TurnquistGreg L. Turnquist has been a software professional since 1997. In 2002, he joined the senior software team that worked on Harris' $3.5 billion FAA telco program, architecting mission-critical enterprise apps while managing a software team. He provided after-hours support to a nation-wide system and is no stranger to midnight failures and software triages. In 2010, he joined the SpringSource division of VMware, which was spun off into Pivotal in 2013.As a test-bitten script junky, Java geek, and JavaScript Padawan, he is a member of the Spring Data team and the lead for Spring Session MongoDB. He has made key contributions to Spring Boot, Spring HATEOAS, and Spring Data REST while also serving as editor-at-large for Spring's Getting Started Guides.
.
Greg wrote technical best sellers Python Testing Cookbook and Learning Spring Boot, First Edition, for Packt Publishing
Facilities
Location
Start date
Start date
About this course
Create, deploy, and run a Spring MVC project using the Spring Tool Suite
Write new Spring MVC controllers and views
Develop an end-to-end interactive web application with forms, web pages, and CRUD functionality
Implement error handling and custom error pages in addition to adding locale support and themes
Create a login page and validation for user registration
Implement shopping cart and ordering functionality
Design a RESTful API and develop exception handling for REST web services.
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 4 years
Subjects
- Team Training
- Writing
- Project
- Web
- Teaching
- Database training
- Database
- Works
- JSP
- Javascript
- HTML
- Server
- XML
- Java
- JSP training
- Javascript training
- XML training
- Access
Course programme
- Downloading an IDE from springsource.org
- Starting the tc server
- Accessing the home page to verify that the server works
- Setting up the development environment
- Creating a new Spring MVC project
- Deploying our project on the server
- Accessing the test page to verify that the application is deployed
- Writing our first Spring MVC application
- Creating a new Spring MVC project
- Importing and building a sample code project
- Adding dependencies for the imported project to the main pom.xml
- Using the startup code
- Understanding the classes and methods
- Including the Spring application context XML in the root context
- Making a call to the business service from the controller
- Saving the result in the model
- Displaying the results on the JSP page by accessing the model map
- Creating a new Controller class
- Annotating the required mappings
- Creating a JSP and redirecting it to the JSP path
- Creating an HTML form in the JSP page
- Adding a new method in the controller and using RequestMapping to map to the form submit
- Saving the object using business services and displaying a success message
- Creating a new controller method to get user data
- Mapping path variables to get the user ID
- Using redirect to redirect from one controller to another, instead of a JSP page
- Adding validation annotations to the model class
- Using the @Valid annotation to have Spring MVC perform validation and redirect the control back to the form in case of errors
- Using Spring MVC's form and error tags to display error messages on the page
- Adding a controller method to fetch user information
- Setting the user information as a model attribute and redirecting the user to the edit JSP
- Wiring the form submit to a new controller method that merges the user's data
- Creating controllers for loading forms
- Adding calls to business services to get related entities to show as dropdowns for users to choose
- Setting the related entities in the model and accessing them from the JSP using the Spring MVC select tags
- Adding theme style sheets and referring to them from the header JSP
- Using ThemeSource to build up a source of themes with unique names
- Using ThemeChangeInterceptors and CookieThemeResolvers to enable users to change themes based on their preference
- Externalizing static text into property files
- Adding Spring MVC's LocaleSource bean to build up locales
- Using Spring MVC's message tags to dynamically look up property values in JSPs
- Adding a category list to the home page
- Implementing the User ModelAndView construct to manage both the model and view
- Using the @ModelAttribute annotation to fetch the required data to display the web pages
- Adding a search page with a search form
- Creating a SearchController that handles search requests
- Using controller method arguments with BindingResult to manage data binding
- Writing a custom Validator class
- Adding the InitBinder method to bind the validator
- Using the @Valid annotation to validate the method argument
- Implementing a method with @ExceptionHandler
- Implementing HandlerExceptionResolver for GlobalExceptionHandler
- Writing an error JSP page to display user-friendly error messages
- Creating a controller and a JSP to display a login form
- Adding a controller method to handle authentication
- Using the HTTPSession method parameter to persist the logged-in user's information in the session
- Building a Cart class with the session scope
- Injecting the Cart instance into the Shopping cart controller
- Adding products to the Cart instance when the user clicks on them
- Building a view cart controller and a JSP page that displays information from the session-scoped cart bean
- Building a "Place order" form and a controller to let users submit the order
- Using flash variables to display messages after redirect
- Writing an Interceptor class by implementing HandlerInterceptor
- Configuring the interceptor in servlet context XML file and mapping URLs
- Extending HandlerInterceptorAdapter to write only the methods you need
- Adding a FreemarkerViewResolver bean definition
- Creating the FTL file
- Creating a controller method that returns the FTL view
- Adding a tiles view resolver definition
- Creating tiles XML and layout pages
- Ordering the view resolver so that the tiles resolver is first in the chain
- Building support for XML and JSON by writing view resolvers
- Including the necessary dependencies and making changes to the entity to make them marshallable
- Adding ContentnegotiatingViewResolver as the first view resolver in the chain
- Understanding the resource URIs and collection URIs
- Understanding HTTP methods
- Understanding the concept of HATEOAS
- Understanding RESTful web services
- Returning a resource instance from the controller method
- Using ResponseBody annotation to indicate that the instance must be converted to the response payload
- Creating RestBean classes to customize the payload format
- Implementing your first REST endpoint
- Using the HTTP methods GET, POST, PUT, and DELETE to map different CRUD methods
- Using the RequestBody annotation to get payload from the request
- Using the ResponseStatus annotation to configure the HTTP response code returned
- Implementing CRUD APIs
- Using the ExceptionHandler annotation to map Controller methods that return the message payloads
- Adding a resource link in the HTTP header by creating an API response
- Using the HATEOAS library to build URIs by directly specifying Controllers
- Implementing Exception handling and HATEOAS
- Downloading an IDE from springsource.org
- Starting the tc server
- Accessing the home page to verify that the server works
- Setting up the development environment
- Creating a new Spring MVC project
- Deploying our project on the server
- Accessing the test page to verify that the application is deployed
- Writing our first Spring MVC application
- Creating a new Spring MVC project
- Importing and building a sample code project
- Adding dependencies for the imported project to the main pom.xml
- Using the startup code
- Understanding the classes and methods
- Including the Spring application context XML in the root context
- Making a call to the business service from the controller
- Saving the result in the model
- Displaying the results on the JSP page by accessing the model map
- Creating a new Controller class
- Annotating the required mappings
- Creating a JSP and redirecting it to the JSP path
Additional information
Learning Path: Spring and Spring Boot Projects