Mastering Haskell Programming
Course
Online
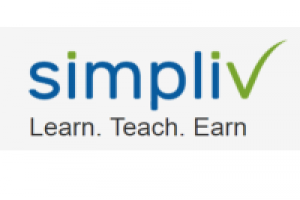
Description
-
Type
Course
-
Methodology
Online
-
Start date
Different dates available
Harness the power of functional programming with advanced Haskell conceptsHaskell is a lazy, purely-functional programming language with a very precise type system. Each of these features make Haskell quite different from mainstream object-oriented programming languages, which is where Haskell's appeal and its difficulty lie.In this course, you’ll discover different ways to structure interactions between the program and the outside world. We’ll look at some subtler aspects of the IO monad, such as lazy IO and unsafePerformIO. In addition to the IO monad, we’ll also check out two other structured forms of interaction: streaming libraries and functional reactive programming.Then we explore parallel, concurrent, and distributed programming. Thanks to purity, Haskell is especially well-suited for the first two, and so there are a number of approaches to cover. As for distributed programming, we focus on the idea of splitting a large monolithic program into smaller microservices, asking whether doing so is a good idea. We’ll also consider a different way of interacting with other microservices, and explore an alternative to microservices.By the end of this course, you’ll have an in-depth knowledge of various aspects of Haskell, allowing you to make the most of functional programming in Haskell.About the AuthorSamuel Gélineau is a Haskell developer with more than 10 years of experience in Haskell Programming. He has been blogging about Haskell for about the same time. He has given many talks at Montreal’s Haskell Meetup, and is now co-organizer. Samuel is a big fan of functional programming, and spends an enormous amount of time answering Haskell questions on the Haskell subreddit, and as a result has a good idea of the kind of questions people have about Haskell, and has learned how to answer those questions clearly, even when the details are complicated. Apart from Haskell, he is a fan of Elm, Agda, and Idris, and also Rust.
Facilities
Location
Start date
Start date
About this course
Find out how to structure larger Haskell programs
Use the Parsec library to easily parse structured inputs
Get acquainted with the process of writing an interpreter, a type checker, and a compiler
See how to model your problem domain with precise types and how to reap the benefits of doing so
Work with Deterministic Communication using IVars and Deterministic Collaboration using LVars
Understand how to do concurrent and distributed programming in Haskell
Know how to cope with the uncertainties of communication in a distributed application
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 4 years
Subjects
- Testing
- Installation
- Semantics
- Programming
- Install
- Programme Planning
- Programming Application
- Information Systems
- Information Visualisation
- IT
Course programme
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- UseunsafePerformIO to inspect when and whether pure code is executed
- UseunsafePerformIO to define global variables
- Learn how unsafePerformIO can accidentally create bugs which are difficult to understand
- Introducing unsafeInterleaveIO by contrasting it with unsafePerformIO
- Introducing lazy IO by contrasting it with ordinary, strict IO
- Introducing effectful stream transformations by contrasting them with pure stream transformations
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- UseunsafePerformIO to inspect when and whether pure code is executed
- UseunsafePerformIO to define global variables
- Learn how unsafePerformIO can accidentally create bugs which are difficult to understand
- Introducing unsafeInterleaveIO by contrasting it with unsafePerformIO
- Introducing lazy IO by contrasting it with ordinary, strict IO
- Introducing effectful stream transformations by contrasting them with pure stream transformations
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Install Stack, which in turn installs the Glasgow Haskell Compiler and all the libraries you will need in order to execute the code from my slides
- Fetch the slides using git and navigate them using git commands
- (optional) Install git-slides to navigate the slides more easily
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- Call C functions from IO, this is where most of the side-effects come from
- Mutate single-cells and arrays from IO
- Create pointers with various semantics supported by the language runtime
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A brief overview of the IO half of the exception handling API
- Learn how to guard resources in the presence of exceptions
- The other half: throwing exceptions from pure code
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- A simple module-based implementation of an effect-tracking monad
- A list-based implementation which simplifies testing
- Use a free monad to allow our implementation to support more complex computations
- Introducing unsafeInterleaveIO by contrasting it with unsafePerformIO
- Introducing lazy IO by contrasting it with ordinary, strict IO
- Introducing effectful stream transformations by contrasting them with pure stream transformations
Additional information
Mastering Haskell Programming