Learning Path: JavaScript: Reactive and Functional JavaScript
Course
Online
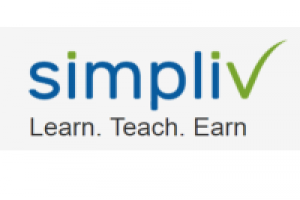
Description
-
Type
Course
-
Methodology
Online
-
Start date
Different dates available
Make your JavaScript code efficient, maintainable, and scalable using the best practices of reactive and functional programmingAlthough JavaScript has many diverse applications, it has become ‘the programming language for the Web’ in the recent times. Almost always, when you see something working smoothly and interactively on the Web, you can assume that there is some JavaScript code running in the background.Packt’s Video Learning Paths are a series of individual video products put together in a logical and stepwise manner such that each video builds on the skills learned in the video before it.JavaScript: Reactive and Functional JavaScript begins with describing what JavaScript is and how browsers use it. We get started with the essentials—the JavaScript syntax. This includes comments, operators, variables, conditionals, loops, and functions. With the basics in place, we move on to learn about reactive programming, understand its need, and also look at building simple apps with good understanding of Rx. We will start off with Bacon.js and then move on to using Rx.js for both client-side and server-side applications. The final part of this Learning Path will look at writing maintainable code. You will experience the benefits of functional programming even if your code is not purely functional. You will also learn how to write code that's easy to understand, extend, test, and debug. Hands-on practice on how to use currying, partial evaluation, map, reduce, filter, recursion, and other functional programming concepts in ES6 is also covered.By the end of this course, you will be able to optimize your JavaScript code.This course is authored by some of the best instructors in this field:About the Author Chip Lambert has been developing websites and web applications for almost 20 years. He is currently a software engineer for Jenzabar Inc. and an online instructor for Bluefield College, teaching courses in web and mobile application development.
Facilities
Location
Start date
Start date
About this course
Learn JavaScript's basic syntax
Discover the Document Object Model and how to manipulate it with JavaScript and jQuery
Change CSS classes on HTML elements on-the-fly
Introduction to reactive programming + Rx in depth
Create operators and perform various operations such as transform, filter, combine, and error handling
Discover the principles of functional programming
Write elegant code with chaining and context binding
Translate SQL queries into chained map and reduce calls
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 4 years
Subjects
- Programming
- Web
- Syntax
- Javascript
- HTML
- Javascript training
Course programme
- We first need to learn the background of JavaScript.
- We then need to choose the software we need to use.
- We did it! We wrote our first simple JavaScript script.
- You must learn how to call JavaScript from the browser.
- Now, you’ll learn the words you can’t use in variables.
- You have learned the basic syntax of the language.
- You need to know what a variable is and the types.
- You must now learn the correct naming conventions.
- Now you know how to declare and use variables.
- Like variables, you must learn what a function is.
- Now, you will learn how to declare and call functions.
- To wrap up functions, you will now learn how to pass parameters.
- We will start by learning what loops are in programming.
- You will then learn the syntax of the for loop.
- We can now execute our code a certain number of times.
- You will learn the structure of the while loop
- You will now learn the do while loop alternative.
- We now have three options for loops and know when to use them.
- You must learn about conditional branching first.
- Now we look at the if statement itself.
- We can now have our code execute only when a condition is true.
- We will look at the switch statement.
- We will now look at an alternative: the if else if statement
- We can use all the available conditional branching statements
- We start by learning what the DOM is.
- You must now learn what the DOM is not.
- Younow have a solid understanding of the DOM.
- Youwill learn the three ways of selecting nodes individually.
- You will now learn how to select multiple nodes.
- Now that we can select nodes, we are ready to manipulate them.
- We need to add an element when needed.
- Times will come when we need to edit items as well.
- We can now add, edit, and delete from the DOM!
- You first need to learn the background of jQuery.
- Now that we know what it is, why should we use it?
- We know the power of jQuery; it is time to unleash it!
- You need to learn how to use it locally.
- Next, you need to learn how to use it via CDN.
- You have now learned both the usage methods and when to use them.
- You must learn the syntax jQuery uses.
- You now need to learn the best place in your script to call it.
- The power and ease of jQuery is starting to come through.
- First, you will learn the syntax of how jQuery does selections.
- You will now learn how to get the HTML or text of an element.
- It is so much easier doing selections with jQuery!
- First we need to change the HTML elements.
- Next, you will learn how to manipulate text within the DOM elements.
- We can now change any HTML element or text we want.
- You need to learn how to add elements before another element.
- Now we need to add elements and text within another element.
- We can now fully manipulate the DOM with jQuery!
- First, you learn about jQuery plugins.
- Now, you learn about the jQuery Validate plugin
- We can now validate HTML forms and get the correct information!
- You will learn about .css() and how to change the CSS
- Now, we will add jQuery effects to an element
- We can now fully manipulate any DOM element
- First, you will learn two different ways to attach events to a DOM element
- Next, you will learn about the jQuery event object and the additional information it provides
- We can now use jQuery events with confidence!
- We first need to learn the background of JavaScript.
- We then need to choose the software we need to use.
- We did it! We wrote our first simple JavaScript script.
- You must learn how to call JavaScript from the browser.
- Now, you’ll learn the words you can’t use in variables.
- You have learned the basic syntax of the language.
- You need to know what a variable is and the types.
- You must now learn the correct naming conventions.
- Now you know how to declare and use variables.
- Like variables, you must learn what a function is.
- Now, you will learn how to declare and call functions.
- To wrap up functions, you will now learn how to pass parameters.
- We will start by learning what loops are in programming.
- You will then learn the syntax of the for loop.
- We can now execute our code a certain number of times.
- You will learn the structure of the while loop
- You will now learn the do while loop alternative.
- We now have three options for loops and know when to use them.
- You must learn about conditional branching first.
- Now we look at the if statement itself.
- We can now have our code execute only when a condition is true.
- We will look at the switch statement.
- We will now look at an alternative: the if else if statement
- We can use all the available conditional branching statements
- We start by learning what the DOM is.
- You must now learn what the DOM is not.
- Younow have a solid understanding of the DOM.
- Youwill learn the three ways of selecting nodes individually.
- You will now learn how to select multiple nodes.
- Now that we can select nodes, we are ready to manipulate them.
- We need to add an element when needed.
- Times will come when we need to edit items as well.
- We can now add, edit, and delete from the DOM!
- You first need to learn the background of jQuery.
- Now that we know what it is, why should we use it?
- We know the power of jQuery; it is time to unleash it!
- You need to learn how to use it locally.
- Next, you need to learn how to use it via CDN.
- You have now learned both the usage methods and when to use them.
- You must learn the syntax jQuery uses.
- You now need to learn the best place in your script to call it.
- The power and ease of jQuery is starting to come through.
- First, you will learn the syntax of how jQuery does selections.
- You will now learn how to get the HTML or text of an element.
- It is so much easier doing selections with jQuery!
- First we need to change the HTML elements.
- Next, you will learn how to manipulate text within the DOM elements.
- We can now change any HTML element or text we want.
- You need to learn how to add elements before another element.
- Now we need to add elements and text within another element.
- We can now fully manipulate the DOM with jQuery!
- First, you learn about jQuery plugins.
- Now, you learn about the jQuery Validate plugin
- We can now validate HTML forms and get the correct information!
- You will learn about .css() and how to change the CSS
- Now, we will add jQuery effects to an element
- We can now fully manipulate any DOM element
- First, you will learn two different ways to attach events to a DOM element
- Next, you will learn about the jQuery event object and the additional information it provides
- We can now use jQuery events with confidence!
- We first need to learn the background of JavaScript.
- We then need to choose the software we need to use.
- We did it! We wrote our first simple JavaScript script.
- We first need to learn the background of JavaScript.
- We then need to choose the software we need to use.
- We did it! We wrote our first simple JavaScript script.
- We first need to learn the background of JavaScript.
- We then need to choose the software we need to use.
- We did it! We wrote our first simple JavaScript script certain number of...
Additional information
Learning Path: JavaScript: Reactive and Functional JavaScript