Learning Path: Learn Functional Programming with JavaScript
Course
Online
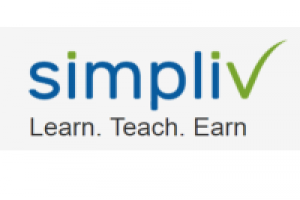
Description
-
Type
Course
-
Methodology
Online
-
Start date
Different dates available
Become a functional programmer by building and testing web applicationsFunctional programming has been around for decades, but it only got adopted by the JavaScript community in recent years. The benefits of using functions as the building blocks of a language are manifold, and when it comes to JavaScript, the advantages are only exponential.This Video Learning Path delivers the building blocks of the functional paradigm in a way that makes sense to JavaScript developers. We’ll look at animated visualizations that’ll help explain difficult concepts such as higher-order functions, lenses and persistent data, partial application, currying, ES6, asynchronous code with promises, and ES2017 async/await.While we anchor these techniques into your mind with the practical usage, you will also learn about techniques to write maintainable software, test-driven development, top-down design, and bottom-up design. Finally, we will use Mocha and Chai to write unit tests for the functional part of the applications.By the end of this Video Learning Path, you will get a hands-on functional application development experience.For this course, we have combined the best works of this esteemed author:Michael Rosata has been a professional JavaScript Developer for 4 years now. He started building web pages. He has worked on a couple of large web apps using JavaScript as well as Apache Cordova. He loves the JavaScript ecosystem and the web community and adopted functional programming as his passion.Zsolt Nagy is a web development team lead, mentor, and software engineer living in Berlin, Germany. As a software engineer, he continuously challenges himself to stick to the highest possible standards when improving his own knowledge. The best way of learning is to create a meaningful product on the way.
Facilities
Location
Start date
Start date
About this course
Understand pure functions and how to refactor impure functions
Work with nested immutable data with lenses using Ramda
Write pure functions to model the DOM and then drop JSX on top of it
Build JSX & Virtual DOM into functional ES2017 apps without using React
See how to rewrite nested asynchronous callbacks with generator functions in a linear fashion
Understand how to model and use infinite sequences with lazy evaluation
Unit test your functional code with Mocha and Chai using test-driven development
Understand the theoretical background of wrapped sets in jQuery, the map function, and flatMap
Reviews
This centre's achievements
All courses are up to date
The average rating is higher than 3.7
More than 50 reviews in the last 12 months
This centre has featured on Emagister for 4 years
Subjects
- Programming
- Writing
- Project
- Web
- Rendering
- Design
- Works
- Javascript
- Javascript training
- Benefits
Course programme
- A pure function does not change values outside of itself
- Given the same inputs, a pure function returns the same output
- Pure functions have a unique mappings from input to output
- Running pure functions inline is representative of a unique value
- Pure functions calling other pure functions won't complicate our mental model
- Identify which parts of our program is pure and which are impure
- Pure functions can return impure functions without breaking referential transparency
- We can defer impure operations and pass results into pure functions as dependencies
- Accepting functions as input creates flexible and generic utilities
- Returning a function to create a reusable utility
- Use higher-order functions to configure objects with lots of setup
- Look at imperative loops, identify the parts that change.
- A wrapped function can pass values in as arguments
- Create higher-order utilities that will work over any array
- Visualize the way map and filter work over a collection
- Create higher-order function implementations of map and filter
- Decouple the work and load from our higher-order functions
- Visualize the steps the reduce function takes
- Implement our own higher-order reduce function
- Map and reduce to work on collections of data
- Learn about creating document fragments and building components
- Use a higher-order function as middleware to perform work
- Like Higher-order functions, components are configurable on input and output
- Function arity is the number of parameters a function defines
- JavaScript totally applies functions regardless of number of input values
- Partial application binds values to parameters without running the function
- Custom “partial” utility to improve the logger from section 2
- Use the Ramda “partial” utility to refactor the same function
- Use the Ramda “curry” utility for most flexible partial application
- Learn about function “call”, “apply”, “bind” methods
- Write a utility that can curry passed in functions
- Improve the utility to allow temporary skipping of placeholder parameters
- The “curry” utility can improve normal map, filter, reduce functions
- “curry” can turn regular functions into better transformation functions
- Follow along with some examples demonstrating the power of partial application
- It’s commonplace to group data with functions to modify it
- Object.freeze disallows assignments or reassignments to properties on an object
- Persistent data structures won’t overwrite, alter or remove previous values
- A lens is created with both a setter and getter
- Lenses don’t mutate the objects they focus on
- Lenses can be combined to focus in on nested data
- Stateless components are functions to input data and output elements
- Write pure functions that imitate the structure of our HTML
- A single impure operation can manage every DOM update
- Babel allows us to write JSX inside our files
- Virtual-DOM caches a representation of how the DOM should look
- Virtual-DOM knows which DOM nodes to update by comparing cache
- A pure function does not change values outside of itself
- Given the same inputs, a pure function returns the same output
- Pure functions have a unique mappings from input to output
- Running pure functions inline is representative of a unique value
- Pure functions calling other pure functions won't complicate our mental model
- Identify which parts of our program is pure and which are impure
- Pure functions can return impure functions without breaking referential transparency
- We can defer impure operations and pass results into pure functions as dependencies
- Accepting functions as input creates flexible and generic utilities
- Returning a function to create a reusable utility
- Use higher-order functions to configure objects with lots of setup
- Look at imperative loops, identify the parts that change.
- A wrapped function can pass values in as arguments
- Create higher-order utilities that will work over any array
- Visualize the way map and filter work over a collection
- Create higher-order function implementations of map and filter
- Decouple the work and load from our higher-order functions
- Visualize the steps the reduce function takes
- Implement our own higher-order reduce function
- Map and reduce to work on collections of data
- Learn about creating document fragments and building components
- Use a higher-order function as middleware to perform work
- Like Higher-order functions, components are configurable on input and output
- Function arity is the number of parameters a function defines
- JavaScript totally applies functions regardless of number of input values
- Partial application binds values to parameters without running the function
- Custom “partial” utility to improve the logger from section 2
- Use the Ramda “partial” utility to refactor the same function
- Use the Ramda “curry” utility for most flexible partial application
- Learn about function “call”, “apply”, “bind” methods
- Write a utility that can curry passed in functions
- Improve the utility to allow temporary skipping of placeholder parameters
- The “curry” utility can improve normal map, filter, reduce functions
- “curry” can turn regular functions into better transformation functions
- Follow along with some examples demonstrating the power of partial application
- It’s commonplace to group data with functions to modify it
- Object...
Additional information
Learning Path: Learn Functional Programming with JavaScript